1. Starting a command line window, running programs
Click Start menu Run… and start cmd.exe
! (There are several other ways to start it.) black console window appears. We will work in this window. The up/down arrow keys (↑, ↓) browse through the previously typed commands, use this feature to spare typing.
Starting a program from the console window.
Just type the name of the program and hit Enter. Type:
notepad.exe
The notepad application starts as if it was started from the Start menu.
Windows automatically completes the name of the program with the appropriate extension (.com
, .exe
, .bat
). So the program could be run without typing the.exe
extension. Close Notepad and type:
notepad
Argunents of the programs
Most console applications, and many window applications too, can interpret command line parameters. Try the following line. What happens?
notepad sometext.txt
Any program can have an arbitrary number ofcommand line parameters (command line arguments). Simply type them behind the name of the program separated by spaces.
If a filename containing space character(s) has to be passed as a command line argument, enclose it in double quotes:
notepad "other text.txt"
The path
How did Windows know the location of notepad.exe
file? There is a list of folders to search for executables. To print this list type:
path
2. Directory commands
The cursor is blinking behind the prompt. By default it is built of the current directory and a >
symbol. Prompt means you are to type a command to the OS!
To list the content of the actual directory (folder):
dir
The list contains further folders and files. Except for the root directory of a device (C:\
) a folder (denoted by <DIR>
) named .
and another named ..
can be seen. The .
means the actual directory and ..
means the parent directory. Start a file explorer window, navigate to the same folder and compare the printed list to the list shown by the explorer.
The dir
command can interpret several command line parameters (switches). For help type:
dir /?
Most Windows OS commands give help like this.
Changing the current directory
To change directory use the chdir
command that can be abbreviated as cd
. Change to the parent directory:
cd ..
Change to the root:
cd \
or
cd /
The folder names in the hierarchy are separated by a \ (backslash) in the path. Windows also supports the UNIX style / separator. Windows does not distinguish between UPPERCASE and lowercase letters (notepad, Notepad, NOTEPAD means the same). Other operating systems do distinguish.
Navigate back to your user directory! Replace 111111 by your username:
cd C:\Users\111111\
Creating and deleting folders
To create a new folder type mkdir
or md
:
md clab
To delete a folder type rmdir
or rd
:
rd clab
The folder remains intact if it contains files.
If you type the following command, two folders will be created named „c” and „lab”: md c lab
.
To create a single folder named „c lab” enclose the name in double quotes: md "c lab"
! (You can enclose the filename even if there is no space in it.)
Certain commands, like dir
interpret filenames containing wildcard characters. The following command will only list files having .exe
extension.
cd c:\Windows dir *.exe
The ?
character matches any single character, the *
character matches any number of arbitrary characters in a filename. Like: dir x*e
list files starting with x, ending with e. There can be several wildcards in a name: dir x*py*e
. Using the question mark:
dir C_85?.NLS
.
3. Redirecting input and output
The more
command can be used for several purposes, now we use it to repeat the text typed in. Type:
more
There is no prompt meaning the more
program is running and waiting for input. Type any text and hit Enter.
What happens? You can type several lines. The Ctrl+Z key combination will terminate more
and brings you back to the command line.
Note: more
reads text until end of file then quits. When typing text an end of file signal can be sent from keyboard by typing Ctrl+Z.
Console programs will also terminate when Ctrl+C is pressed.
Type:
more > mytext.txt
Type some lines of text! What happens? Quit more
and list the directory. What happens?
C:\WINDOWS> more > mytext.txt Access denied
This error message is displayed if your current directory is write protected. (Why should not write a user into the folder containing files of the operating system?)
Switch to another folder, if this happens. C:\Users\111111
Type:
more < mytext.txt
What happens now? Note that there was no need to hit Ctrl+Z because more
printed the content of the file mytext.txt
.
Explanation: Console programs communicate with the user via standard input and standard output. By default standard input is the keyboard, standard output is the console screen. Using the above methods they can be redirected. The programs do not even know if they are using the default device or some file.
more >> mytext.txt
will append the newly typed text to the end of the previous content of mytext.txt
(using a single
>
will overwrite any previous content). If mytext.txt
does not exist, both forms will create a new file.
Beside standard output there is a standard error for printing error messages of the program. By default it is connected to the console screen, too. If the standard output is redirected and some error occurs, like more nosuchfile.txt > mytext.txt
, the error message still appears on the screen, instead of the file. To redirect standard error use 2>
:
more nofile 2> error.txt
Ctrl+C and Ctrl+V can not be used for copy/paste in a command window (remember, Ctrl+C quits the program). They can be found in the window menu of the console window. After initiating Mark (under Edit) use the mouse or the arrow keys (with shift for selecting) to select a rectangle. To actually copy it hit Enter. The size and appearence of the console window can be changed from the window menu, too.
Note: if you do not want to type the entire name of an existing file, just type the first few characters and then hit the Tab key. The command line will complete the filename. Like my
Tab has the same effect as typing mytext.txt
. If there are several matching files, consecutive tab hits will cycle through the matching files.
Windows pipe
Type
cd c:/Windows/System32 dir
There were too many files in the list scrolling off the screen just too fast. The real use of more
is to show text page by page on the screen.
Use it to see the complete list:
dir | more
The used OS feature is the pipe: connect the output of a program to the input of another one. Again it is about redirecting input and output.
In the more
program space advances by a page (screenful). To advance a line, press Enter. To exit press Q.
4. The Code::Blocks IDE
An integrated development environment (IDE) helps develop (code editing, building, testing, debugging) programs. As C language is very widely used a lot of such tools exist. The recommended IDE for this subject is a free tool Code::Blocks that is also installed on the computers of the Students' Computer Center.
Start the Code::Blocks program. Choose File menu/New/Project… submenu. Create a new project following the pictures below. Pay attention to the details.
- The type of the project should be
Console application
. ClickGo
. - The next page is to select the language. Of course it is C.
- Then Code::Blocks will let you name your project (e.g. first). In the next line select a folder to create your project in. If it is empty, hit the
...
button and browse to Documents in your home folder. (Remember this path!) Important: neither the folder name, nor the path should contain space or accented letters! - In the fourth window there is usually nothing to change. Compare to the picture.
- Your new project is ready. The files of the project can be seen in the „Management” panel. Opening the „Sources”
folder you can find the main (and only) program module:
main.c
. It contains a Hello World program.
5. Hello World
Code::Blocks created the new project with a simple Hello World program. If you use a different IDE paste this C program (double-clicking in the code selects all the program):
#include <stdio.h> int main(void) { printf("Hello world!\n"); return 0; }
Look at the code in the editor panel of the IDE: it appears in different colours. Which words are coloured? What is the advantage of this feature? Easier to read the code and spot certain mistypings. Many IDEs mark the corresponding pair for the brackets and parentheses.
The executable program
Our own program created by the IDE works the same way as other command line applications: there is an .exe
file, and its output can be redirected. Clicking the „Build and run” (green play + yellow gear symbol) button or selecting the Build/Build and run submenu will run your program. First the code is compiled then the executable is started. The result should look like this.
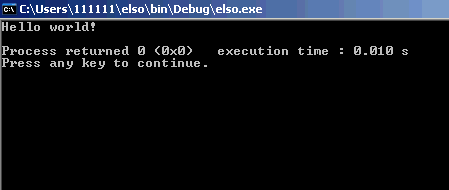
Find the compiled executable on the comuter. It must be in the folder selected at the project creation.
Open a command window (cmd) and navigate (cd) to that folder. Beside main.c
you will see some other files. There will be a folder named bin
and another folder Debug
in it. And in this folder you can see the executable of your first project (first.exe
). Start it!
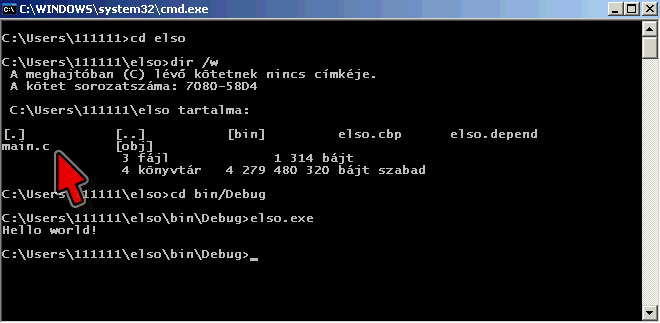
The compiled program is a file that contains machine instructions to print the required text, plus a few other things meaningful for the operating system. This is the executable (frequently called binary). It is not meaningful for humans, look at the content using the type
(cat
under Linux) command.
Hint: if you forgot the name of the folder it is easy to locate it.
Right-click on a file in the „Management/Project” panel of Code::Blocks (like main.c
) and select „Properties…”: at „File name (absolute)” property you will see the file name with full path:
C:\Documents and Settings\Name\Dokumentumok...
– Windows ≤5 (XP).C:\Users\Name\...
– Windows ≥6 (Vista)./home/name/...
– Linux.
Files of the IDE project
Examine the other files in the project folder! Especially main.c
and first.cbp
(this has the name of the actual project). You can open them in notepad(notepad first.cbp
) or simply type/cat/more
them.
The main.c
file contains your program:
#include <stdio.h> int main(void) { printf("Hello world!\n"); return 0; }
The project file first.cbp
contains data and settings of the project, maintained by CodeBlocks:
<?xml version="1.0" encoding="UTF-8" standalone="yes" ?> <CodeBlocks_project_file> <FileVersion major="1" minor="6" /> <Project> <Option title="first" /> <Option pch_mode="2" /> <Option compiler="gcc" /> ...
Note. Windows explorer by default hides file extensions (instead of main.c
you only see main
). This mighty might be advantageous for office workers but it is disastrous for the rest of the world, especially for programmers. You are encouraged to change this behaviour on your own computer.
Redirecting the output
Test output redirection using the >
character for your first program. Redirect output to hello.txt
then print the file using the type
command!
Obviously your program behaves exactly the same way as any other command line program. Redirection is a feature of the operating system and does not require any modification in your program. These programs are called console or command line applications; remember this is the type we selected during the creation of our project.
6. Painting
Task: Write a program that calculates how many cans of paint must be purchased to coat every (outer) surface of a cylindrical container. You must define variables of appropriate type, read in and print out values.
The height and the diameter of the cylinder is known. Yield of the paint: one can of paint is sufficient for a surface area of 2 m2.
Paint the container Height? 2 Diameter? 1.2 4.900885 can(s) of paint needed.
You can use an approximate value of 3.14159265
for Pi. If your program delivers different result re-check your formulae.
Format of the printout
A millionth of a can is not relevant but rather disturbing piece of information. To make the printout human friendly it is wise to round the printed value to one decimal digit following the decimal point: in printf
change %f
to %.1f
.
4.9 can(s) of paint needed.
Solution
#include <stdio.h> int main(void) { double diameter, height; printf("Container painting\n\n"); printf("Enter height: "); scanf("%lf", &height); printf("Enter diameter: "); scanf("%lf", &diameter); double radius = diameter/2; double cans = (2*radius*radius*3.14159265 + height*2*radius*3.14159265) / 2; printf("\nNumber of cans to buy: %f\n", cans); return 0; }
7. \n and %d
Start a Hello World program. Modify the printed text:
printf("Hello\nworld\n\n!");
What happens?
What happens when using two backslash characters \\
?
printf("Hello world!\\n");
Print out the patterns below using one single printf()
statement in each case.
/ / /
\ \ \
printf("hello\n") will print "hello", and starts a new line.
Using "%d" we can print the value of an integer variable.
Solution
#include <stdio.h> int main(void) { /* top left output: */ printf(" /\n /\n/\n"); /* top right output: */ printf("\\\n \\\n \\\n"); /* bottom left output: */ printf("printf(\"hello\\n\") will print \"hello\",\nand starts a new line.\n"); /* bottom right output: */ printf("Using \"%%d\" we can print\nthe value of an integer variable.\n"); return 0; }
8. Further problems for practicing
Task: scanf in C
Create a C program that practices the usage of scanf with the %d format specifier.
The program should follow these steps:
- Declare variables to store the two integers.
- Prompt the user to enter the first integer.
- Read and store the entered value using scanf with %d.
- Prompt the user to enter the second integer.
- Read and store the entered value using scanf with %d.
- Calculate the sum of the two integers.
- Calculate the product of the two integers.
- Display the sum and product using printf. For example:
#include <stdio.h>
int main() {
// Declare variables
// Prompt the user to enter the first integer
// Read and store the first integer using scanf with %d
// Prompt the user to enter the second integer
// Read and store the second integer using scanf with %d
// Calculate the sum of the two integers
// Calculate the product of the two integers
// Display the sum
// Display the product
return 0;
}
Money
Creat a program that asks the user how many 50, 100 and 200 Ft coins does he have in his pocket. The program should then calculate how much money he has.
Celsius–Fahrenheit
Create a program that asks the user a real number, a temperature value in Celsius. The program should print the temperature in Fahrenheit (0°C=32°F, 40°C=104°F, linear)! Write the other way converter, too!