1. Errors in the program
The following code contains some errors. Paste into a new IDE project (replacing the Hello World app).
#include <studio.h>; int main{} { int x; printf("Dear user!"); printf("Enter a number, I will print its square!"); scanf("%d", x); x = x*x printf("x square is: %f", x); }
Try to build, and observe the error messages! Clicking an error message will put the cursor to the corresponding position in the editor pane. Always start with the first error message, try to understand the meaning of the messages! There are 7 errors in this code.
It is important to pay attention also to the warning messages, not just to the errors. There are two kinds of messages:
- Error messages
- Violation of language rules, the program can not be compiled.
- Warnings
- These are not language mistakes. The program can be compiled but the compiler found something suspicious, unusual, probably a mistake. Programs that compile with warning(s) usually do not work as expected.
Solution
Errors in the program:
- The proper include file is called stdio.h instead of studio.h
- No semicolon (;) is needed after the #include
- Instead of main{} the correct solution is main()
- The end-of-line has been forgotten in the first printf
- In case of scanf, the second argument must be preceeded by &
- Semicolon (;) missing in the x=x*x line
- In the last printf, %d should be given instead of %f, since the type of the variable to print is integer
- return 0; is missing at the end of the main
2. Length of a line
Write a program that reads the x
and y
coordinates
of two 2D points (4 numbers to read!) and prints the Euclidean distance of the two points. Use the Pythagorean formula.
Choose an appropriate data type for storing the coordinates.
The calculation involves square root operation. Use the sqrt()
function of the mathematical library of C. It is given in math.h
so the following line must be placed at the beginning of your program:
#include <math.h>
Note: Those compiling the program from a Linux shell must link the mathematical library using the -lm
switch.
line | length |
---|---|
(0;0)–(1;1) | 1.414214
|
(1;5)–(4;1) | 5
|
(-3;2)–(5;7) | 9.433981
|
Solution
#include <stdio.h> #include <math.h> int main(void) { double px, py, qx, qy; double distance; printf("x coordinate of point p: "); scanf("%lf", &px); printf("y coordinate of point p: "); scanf("%lf", &py); printf("x coordinate of point q: "); scanf("%lf", &qx); printf("y coordinate of point q: "); scanf("%lf", &qy); distance = sqrt((px-qx)*(px-qx) + (py-qy)*(py-qy)); printf("Distance between p and q: %f\n", distance); return 0; }
3. Parabolic equation
Write a program that reads the a
, b
and c
parameters of a parabolic (second order) equation
given as ax2+bx+c=0
and prints the
x1
and x2
solutions!
The formula:
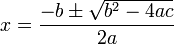
Solve the equations given in the left table with your program. Then try to solve those given in the right table. What happens? Why?
equation | solutions |
---|---|
2x2-x-6=0 | x1=2, x2=-1.5 |
x2-12x+35=0 | x1=5, x2=7 |
equation | solutions |
---|---|
x2-2x+1=0 | ? |
x2+2x+10=0 | ? |
Modify the program to treat those cases when there is one single solution or when there is no solution at all.
Hint: You must examine the value of discriminant BEFORE applying sqrt on a negative value.
Solution
We have to make sure to not to take the square root of negative numbers. Hence, before taking the square root the discriminant has to be examined to determine the number of real roots.
To compute the discriminant we need a realively complex C expression that includes many operators (addition, subtraction, multiplication, etc.). There operators have a specific precedence rules in C as well as they have in mathematics. For instance, multiplication has a higher precedence than addition. The precedence rules of the C programing language are quite complex, we are going to discuss it in details later.
The if (discr==0)
condition, while correct, should never be used in the practice. Due to rounding errors it is possible that an expression
returns a nearly zero number even if the result should be zero theoretically. Again, this issue will be discussed later in the semester.
#include <math.h > #include <stdio.h> int main(void) { double a, b, c; printf("a="); scanf("%lf", &a); printf("b="); scanf("%lf", &b); printf("c="); scanf("%lf", &c); /* the discriminant is: b² - 4ac */ double discr; discr = b * b - 4 * a * c; /* if <0, both roots are complex numbers */ if (discr < 0) { printf("No real roots!\n"); } /* if the discriminant is 0, there is exacty one root */ if (discr == 0) { printf("One real root: %f\n", -b / (2 * a)); } /* if >0, there are two real roots */ if (discr > 0) { printf("Two roots: %f, %f\n", (-b + sqrt(discr)) / (2 * a), (-b - sqrt(discr)) / (2 * a)); } return 0; }
4. Loops: printing numbers
The pseudo-code of a program that prints the numbers from 1 to 20 (below each other) is presented here.
Take a number, say 1. As long as the number ≤ 20 Print the number. Start a new line. Advance the number by 1. End of repetition
C code
Implement this program in C using while()
loop!
Please note: as you type the curly bracket {
after
while()
and hit Enter the editor of the IDE will start the new line to the right by a few spaces. After the closing }
it goes back to the left. Use this feature to make your program more readable: to make it obvious what is inside the loop.
If the formatting goes wrong (by deleting/adding spaces, etc.) Code::Blocks can automatically reformat your code:
right click in the editor pane, select „Format use Astyle” from the context menu. Always keep your code clean, readable and self explanatory!
Tracing, debugging
Try tracing your program! The simplest way to do it in Code::Blocks is to navigate with the text cursor to the line you want to stop program execution and press F4 (Debug/Run to cursor). Now each pressing of F7 (Debug/Next line) will advance execution by one line. The „Debug/Debugging windows/Watches” menu toggles a window in which the actual value of the variables can be seen (Local variables).
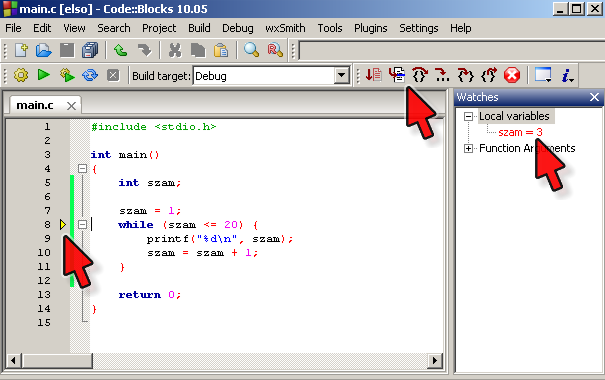
Keep in mind that the condition of the loop controls how long the body should be repeated; thus the loop keeps iterating the body as long as the condition is true. When it gets false, the first statement after the body of the loop is executed. Look at the value of the loop variable in the „Watches” window! What is the value after the loop stops?
Replace the while()
loop with a for()
loop! Follow the execution of this version line by line, too.
Input the bounds
Read in numbers. Modify your program to print values between a lower and an upper bound entered by the user, instead of between 1 to 20. It is not nice to have an empty window with a blinking cursor, print some instructions to the user what we expect from him/her to enter.
Reversed bounds. Test what happens if the user enters the lower and upper bounds reversed
(20–1 instead of 1–20). Debug your program to see what happens!
Modify/complete the program to handle this case.
After reading the two bounds you first have to check which of them is the lower one and set the newly introduced from
and to
variables accordingly. Then use them to run the loop.
5. Line of a given length
Write a program that reads a value from the user and draws
a line built of +
and −
symbols. The number of the −
characters should be equal to the entered value.
Length of the line? 4 +----+
First write the pseudo code of the program using paper then the C source code using the computer.
Hint: no if()
is needed for this program. If you happen to have one try to get rid of it.
There is always a +
at both ends.
Solution
#include <stdio.h> int main(void){ int length; printf("Length of the line: \n"); scanf("%d", &length); /* asking the user to enter the length */ printf("+"); /* + sign at the beginning of the line */ for (int i = 0; i < length; i = i+1) /* A loop printing the - signs */ printf("-"); printf("+"); /* + sign at the end of the line */ return 0; }
6. Further problems for practicing
Square numbers
Write a program that prints the first N square numbers (1,4,9,16,25...)! Read N from the user
Print all square numbers less than N. (This is a different problem.)
Spheres
Print the volume of all spheres, the volume of which is less than 1 cubic meter, starting from a radius of 10 cm, increased by 10 cm each time!